The goal of this guide is to demonstrate how to create a DApp that mints ERC-20 tokens on the Polygon network in as little time as possible using ethers.js and Bunzz without writing Solidity.
This guide is divided into two parts, both covering the following major concepts respectively:
- Creating and deploying an ERC-20 token to the Polygon blockchain in five minutes (no jokes).
- Minting an ERC-20 token using ethers.js
Prerequisites to create and mint an ERC-20 Token on Polygon Network
- Bunzz — to create and deploy our token and smart contract without writing solidity code
- Ethers.js — To interact with the deployed smart contract
- MetaMask — to interact with the Polygon Network
- Quick Node — to interact with Polygon Testnet (also called Polygon Mumbai)
- Some MATIC tokens — we can get them from the faucet
Let’s get started.
Set up QuickNode with Polygon Testnet nodes
What is QuickNode? QuickNode is a platform that helps you access the blockchain environment without the hassle of hosting your own node, saving time and resources.
It lets you access blockchain nodes in a few clicks and you can scale the node performance according to your need thus creating an environment for you to scale your DApp.
- Sign in / Sign up for your QuickNode account
- Click on create a QuickNode endpoint
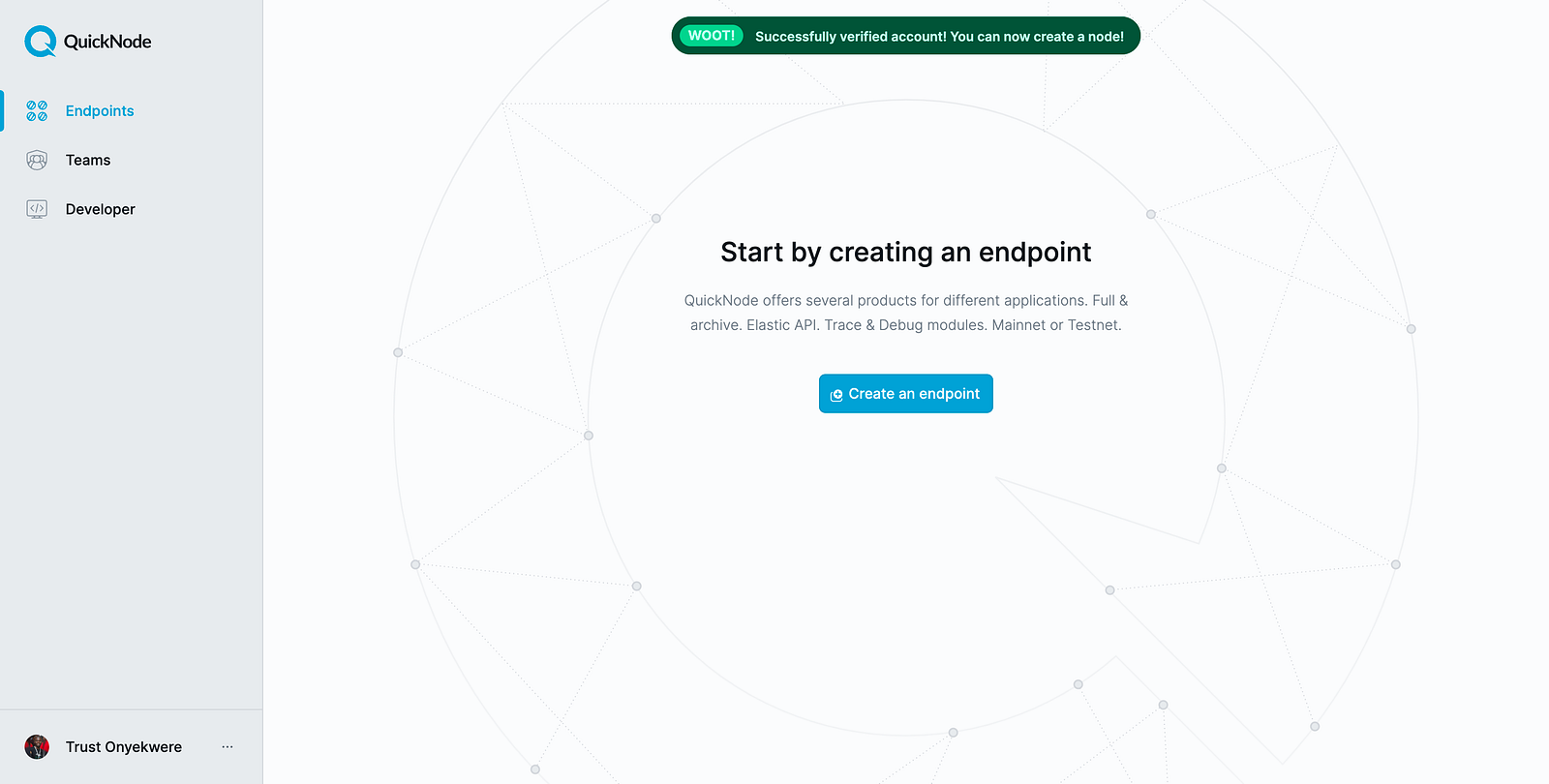
- Now select Polygon as Network and Mumbai Testnet as chain and continue
- We don’t need any add-ons so click Create Endpoint now
Once you create the endpoint you can start setting up our MetaMask Wallet with QuickNode.
- Go to your MetaMask wallet and click on the list of networks
- A drop-down list will show up with a list of networks but you need to click on Add network at the bottom of the list
- Now you will have a form to fill up to add a new network to your MetaMask account
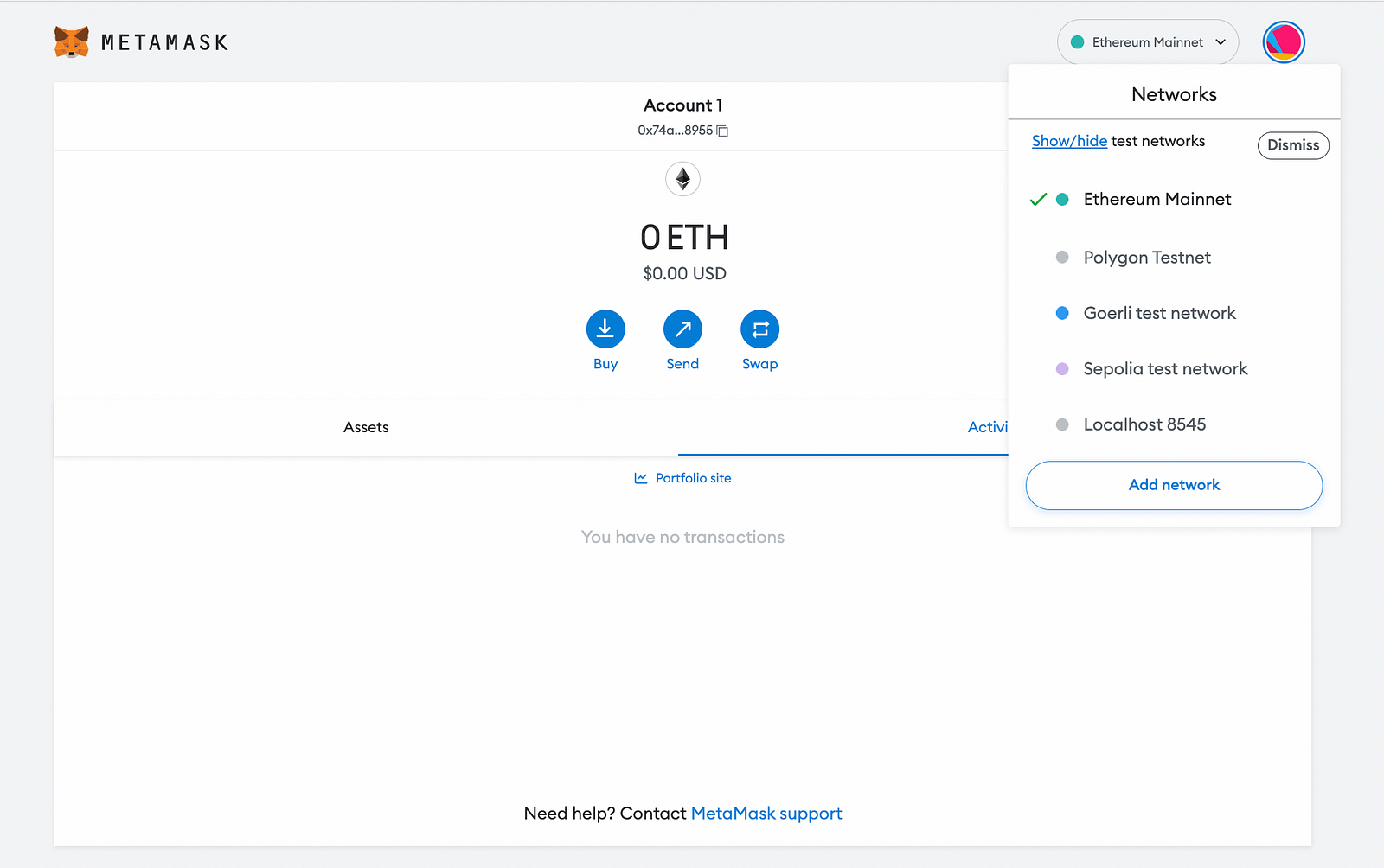
- Go back to QuickNode Endpoint Dashboard, and copy the HTTPS Provider link.
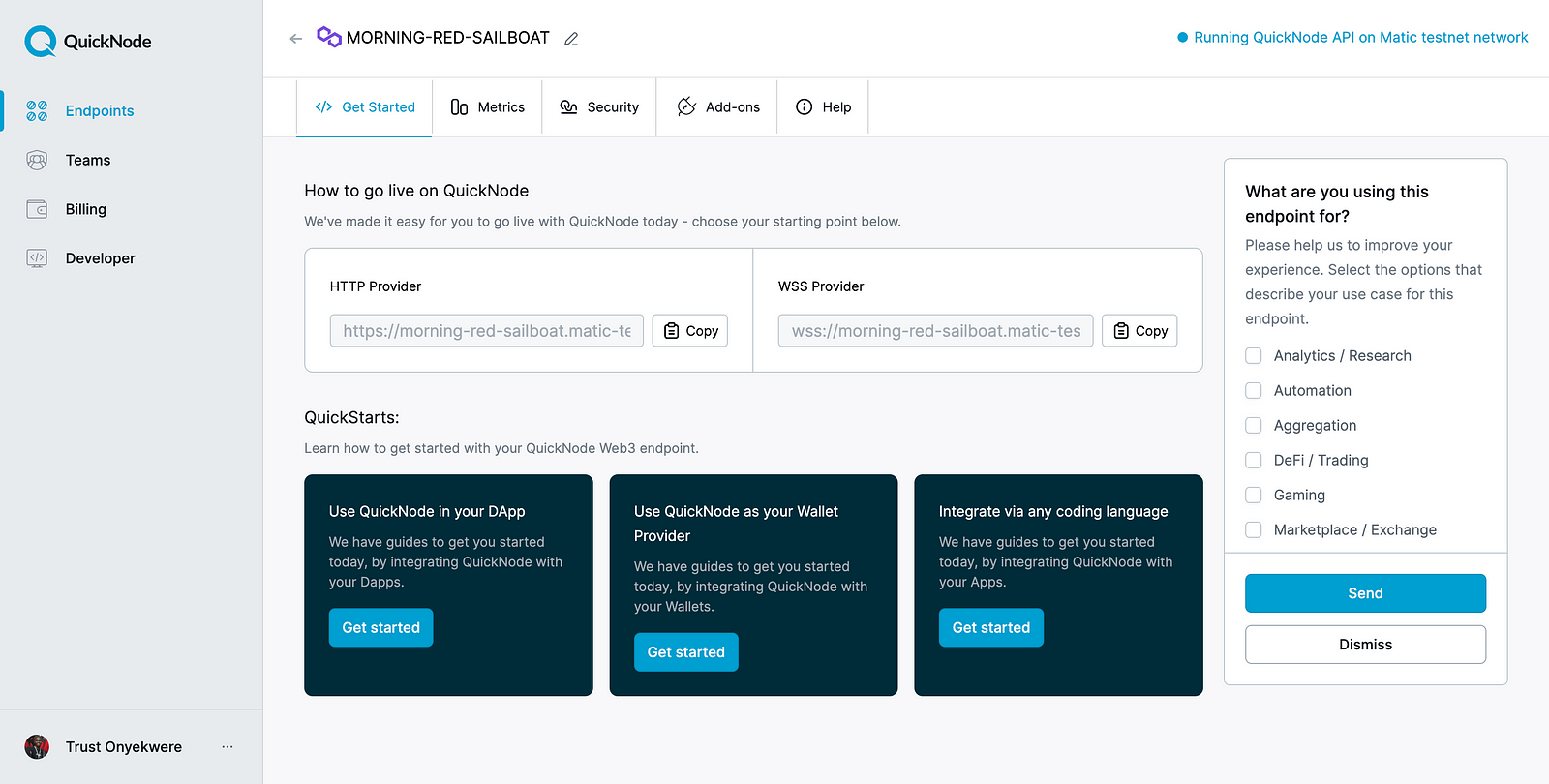
Now let’s go back to the MetaMask add network form and paste the:
- HTTPS in New RPC URL field with 80001 as Chain ID
- “MATIC” as a Currency Symbol
- Polygon Testnet as Network Name (any name you like) and,
- Finally, https://mumbai.polygonscan.com/ is Block Explorer URL
- Done? Click Save
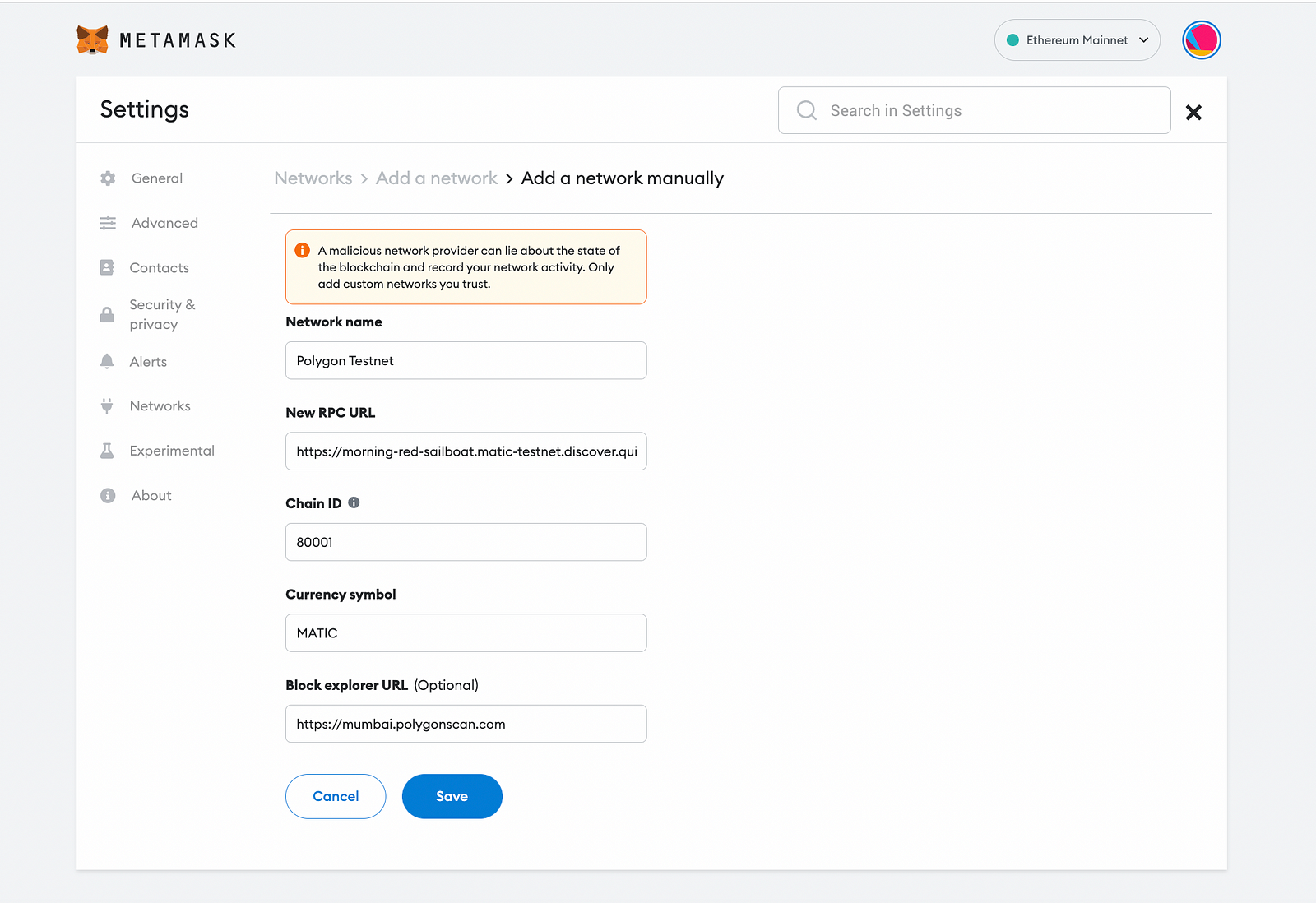
So, we have finished setting up MetaMask for our token and by now you will have created your Polygon Metamask Address. Let’s go get a free MATIC token to pay for the transaction fees.
Let’s get us some free MATIC from polygon Faucet
Since we are on Testnet, we can go to the faucet and get free MATIC.
- Go to https://faucet.polygon.technology/
- Select Mumbai as Network, MATIC as the token, and then paste your MetaMask Polygon Address. Click Submit and confirm the transaction
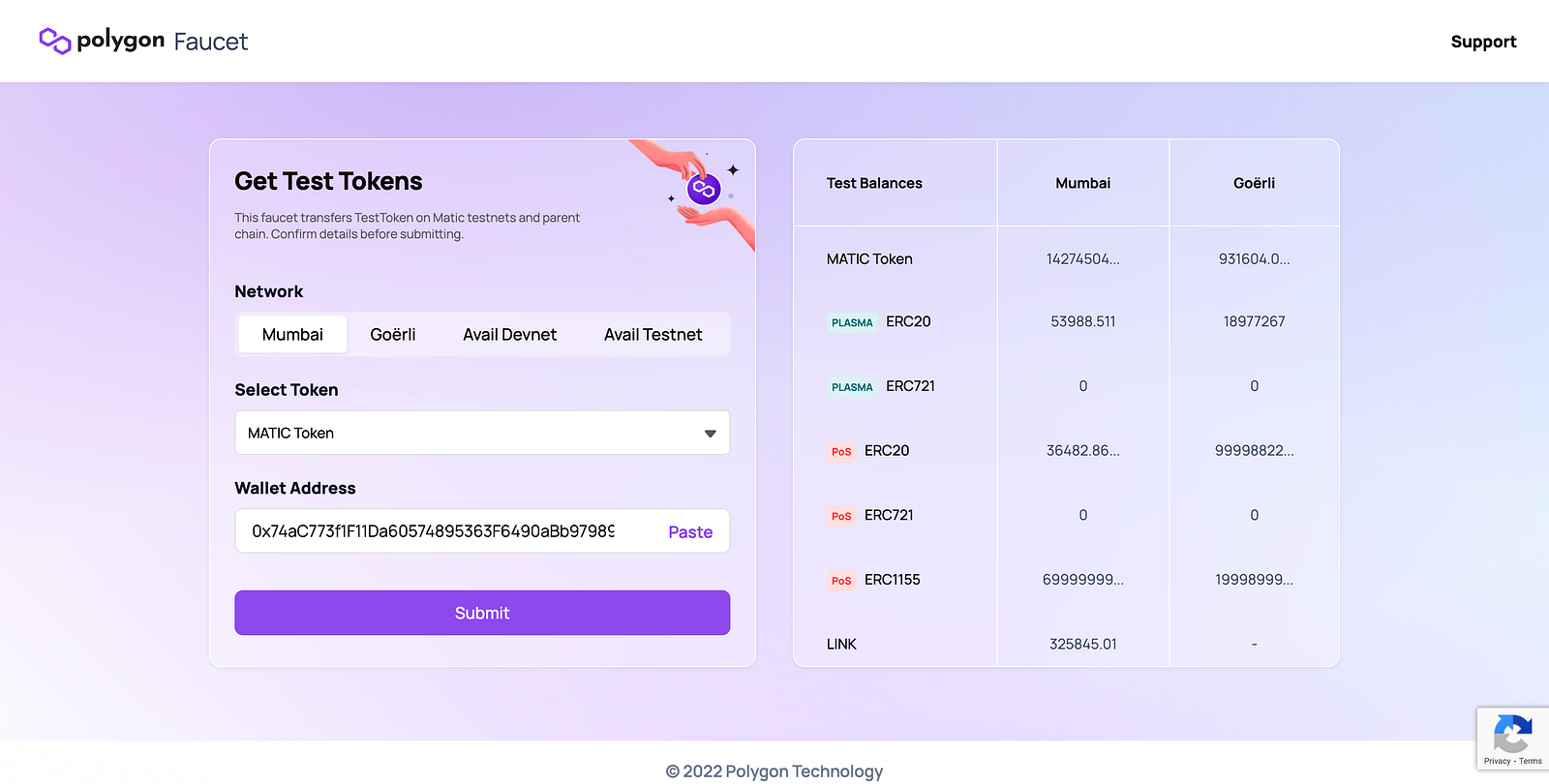
Now you will have some MATIC tokens in your account to pay for the transaction fees.
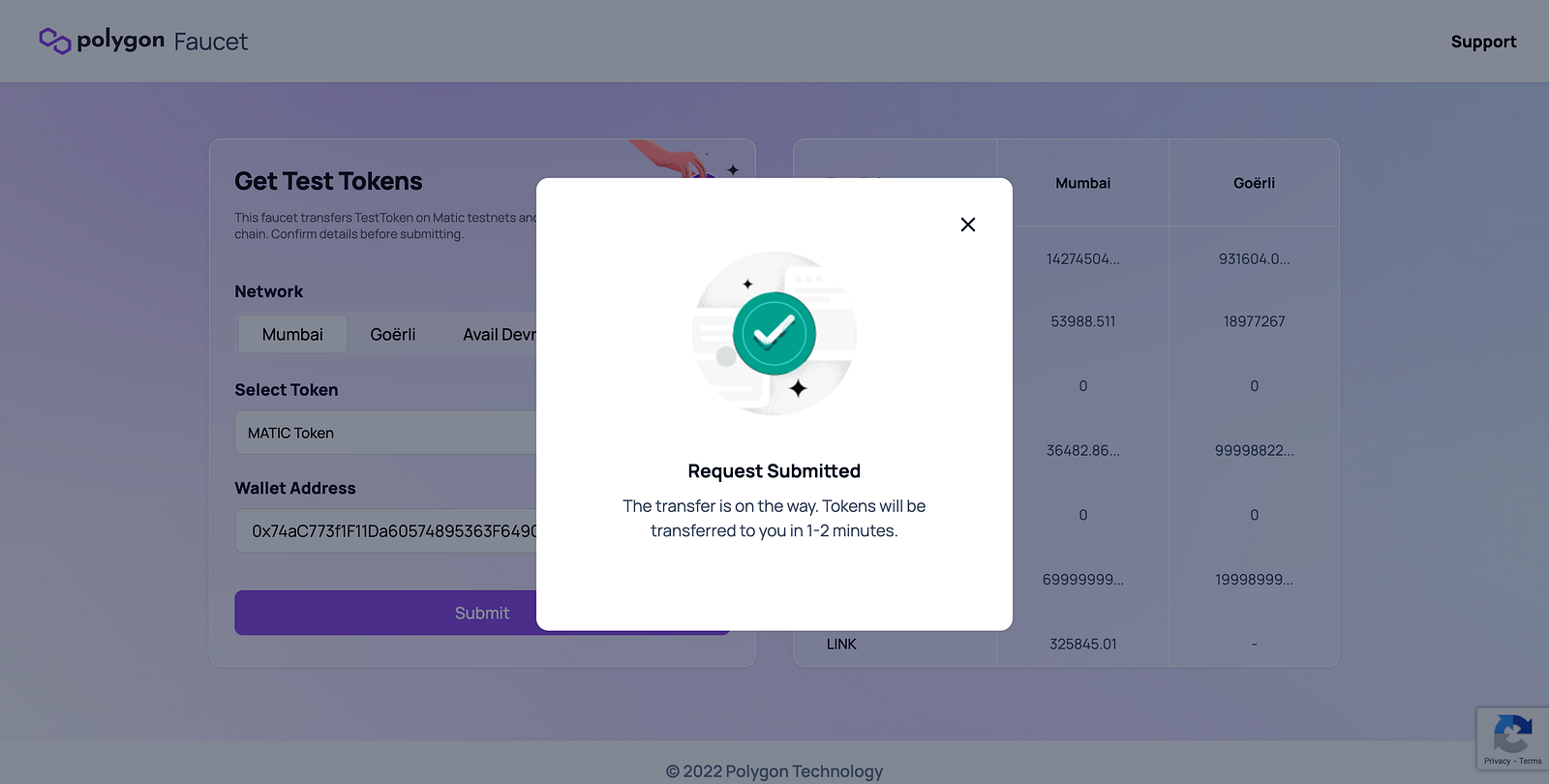
We have just finished setting up everything we need for the deployment.
Next, you will create your own ERC-20 token contract in just 5 minutes without writing solidity code! No joke.
Deployment and token launch on Polygon Testnet
Go to Bunzz and log in or sign up if you’re new to the platform.
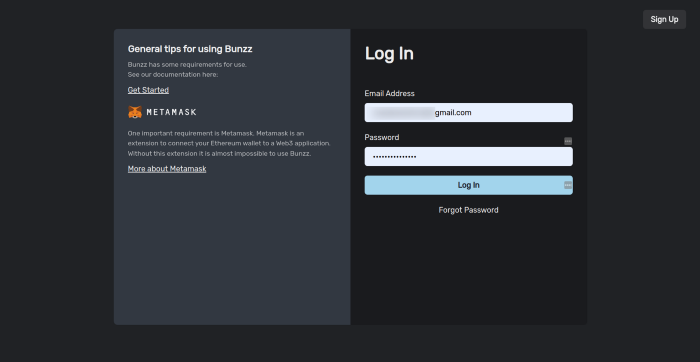
Once you are logged in, you will have access to the Bunzz dashboard where all the magic happens.
- Connect your Metamask wallet and then
- Click on “Create DApp”
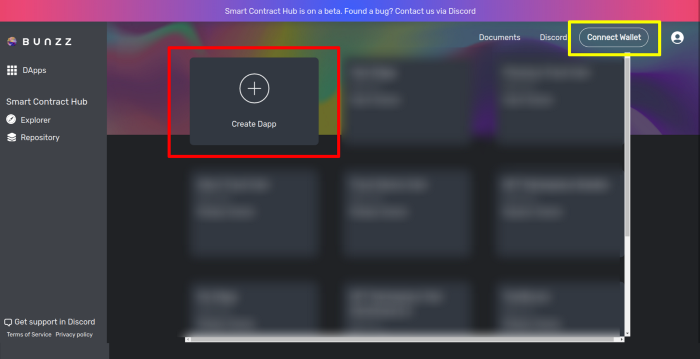
- Give your DApp a name.
You can use any name. I used Milk Token for mine.
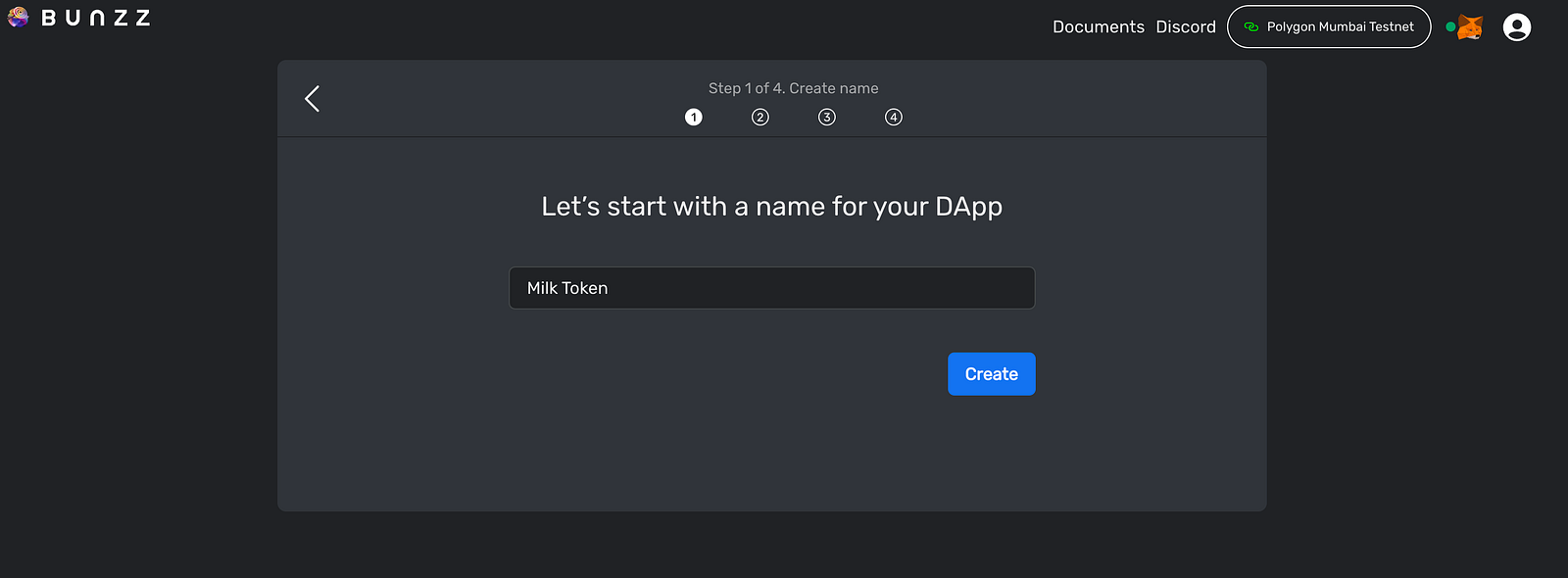
Next, Select a blockchain network for the DApp. Scroll through the options in the dropdown menu and select Polygon Mumbai Testnet.
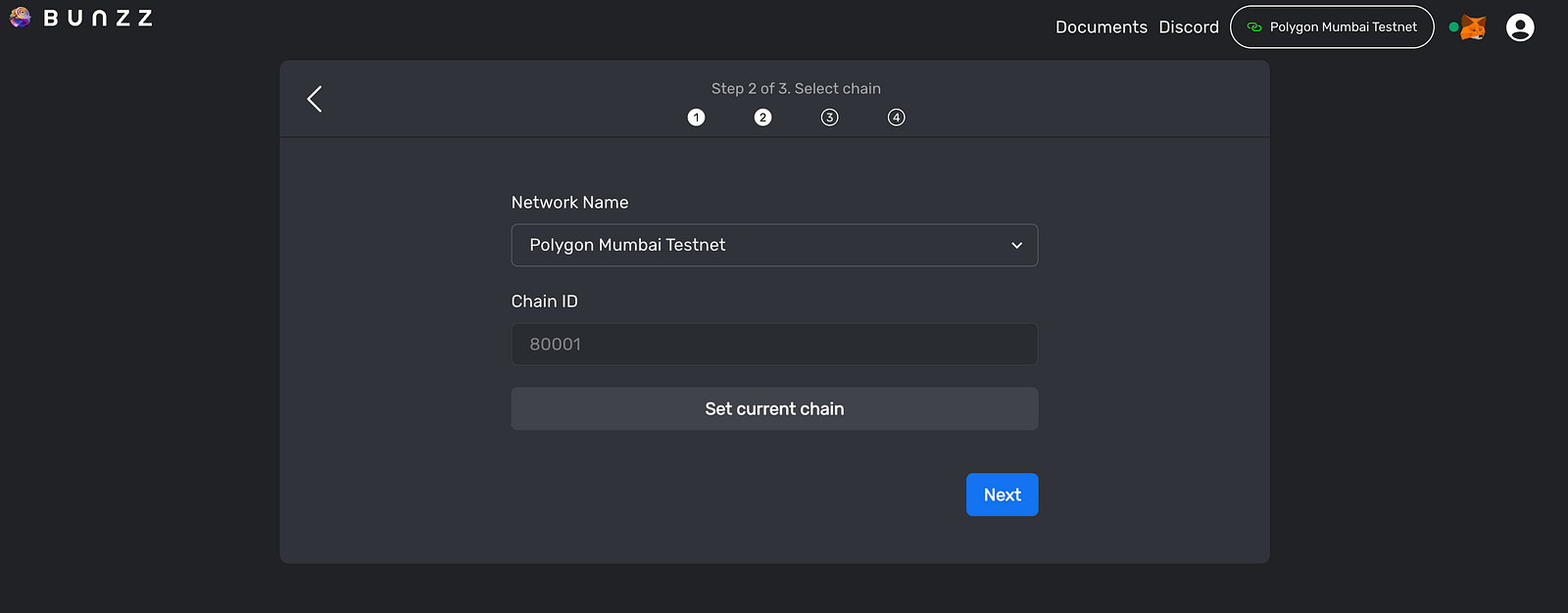
Up next is to select a template from the many available already-made smart contract templates.
- Select the simple ERC20 Token.
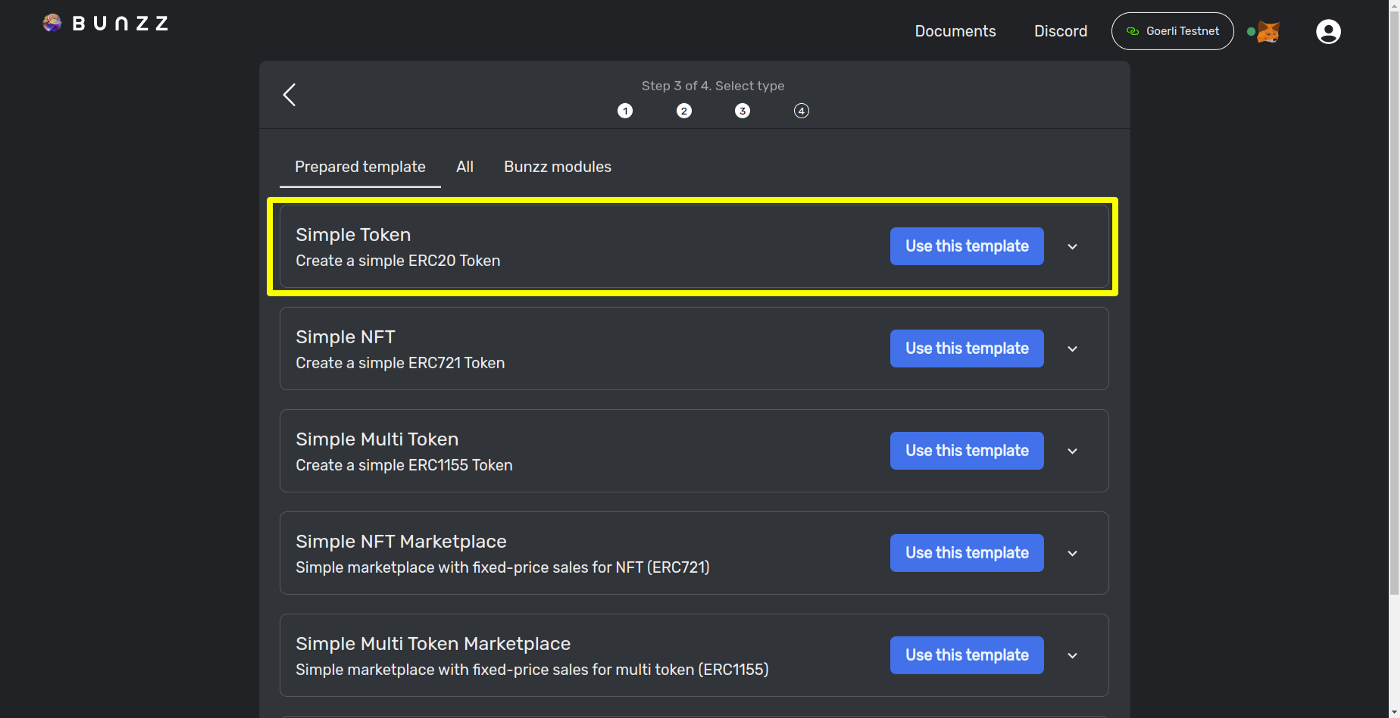
- Customize the token by adding a name and a symbol.
I have named mine Milk Token and MTK as the symbol.
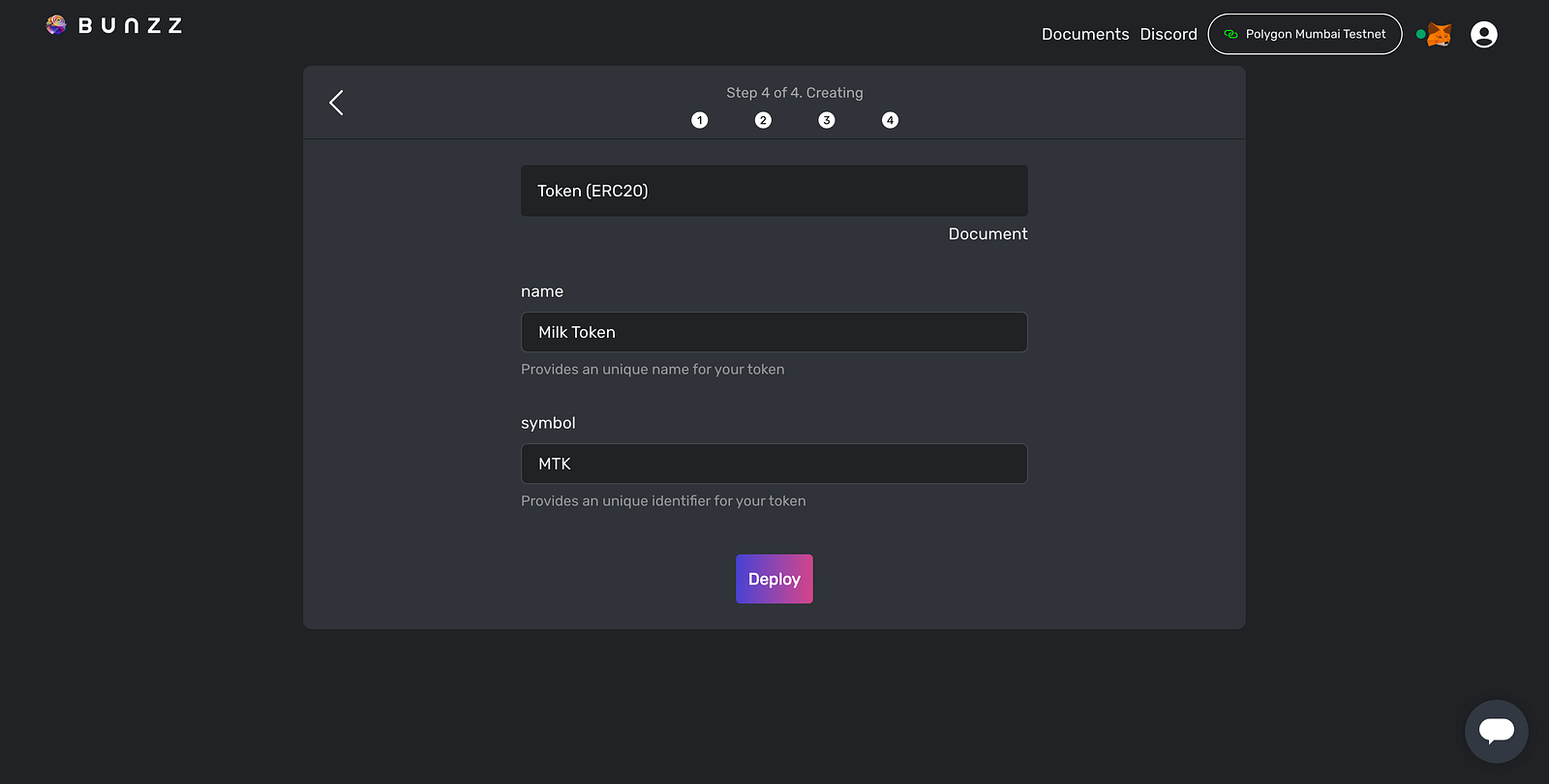
Once you are done customizing,
- Click on deploy and wait for it to sync by approving three transactions that would pop up via Metamask.
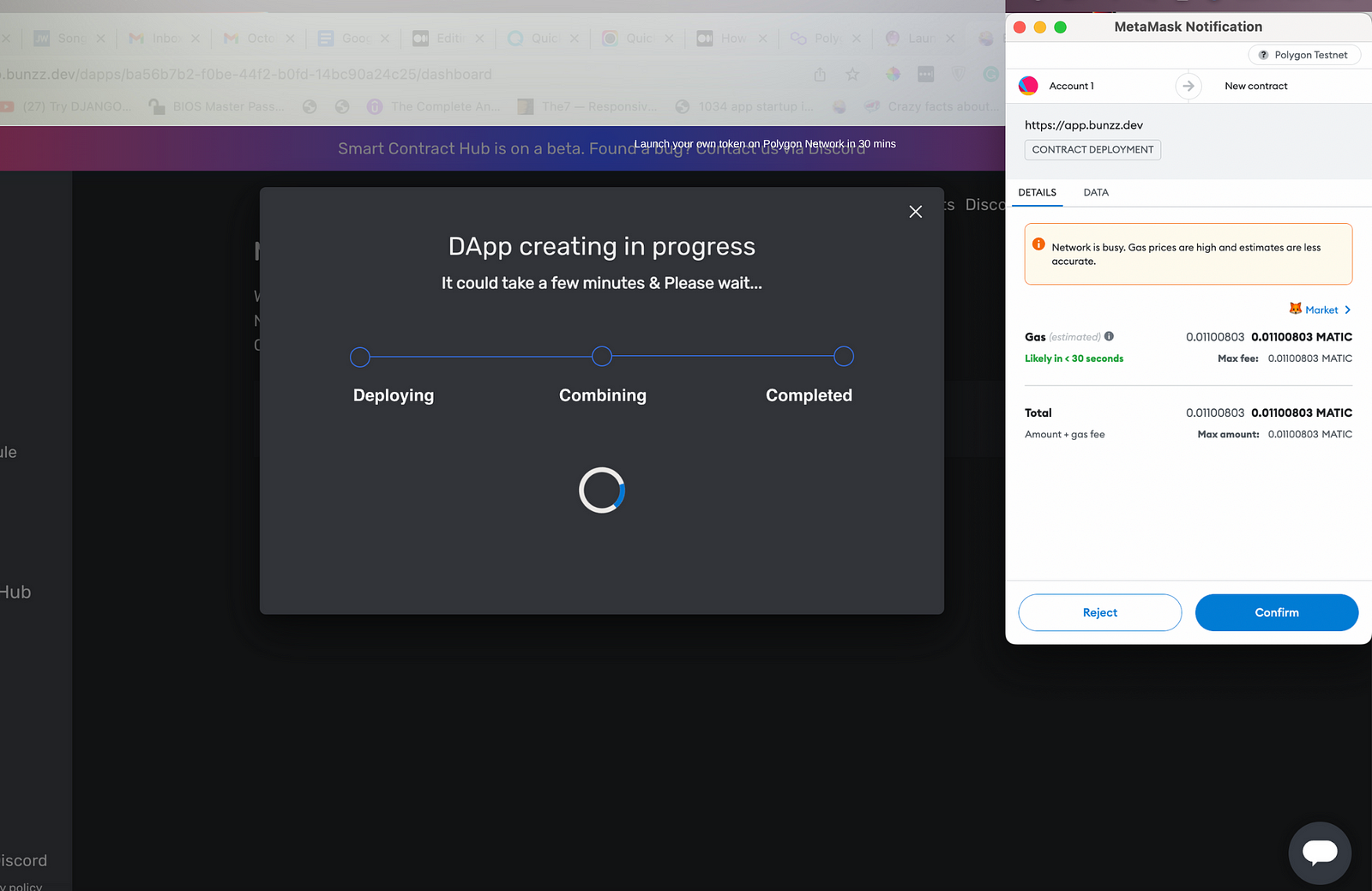
Hurray!
You’ve done it. You have successfully deployed an ERC20 smart contract for our new token.
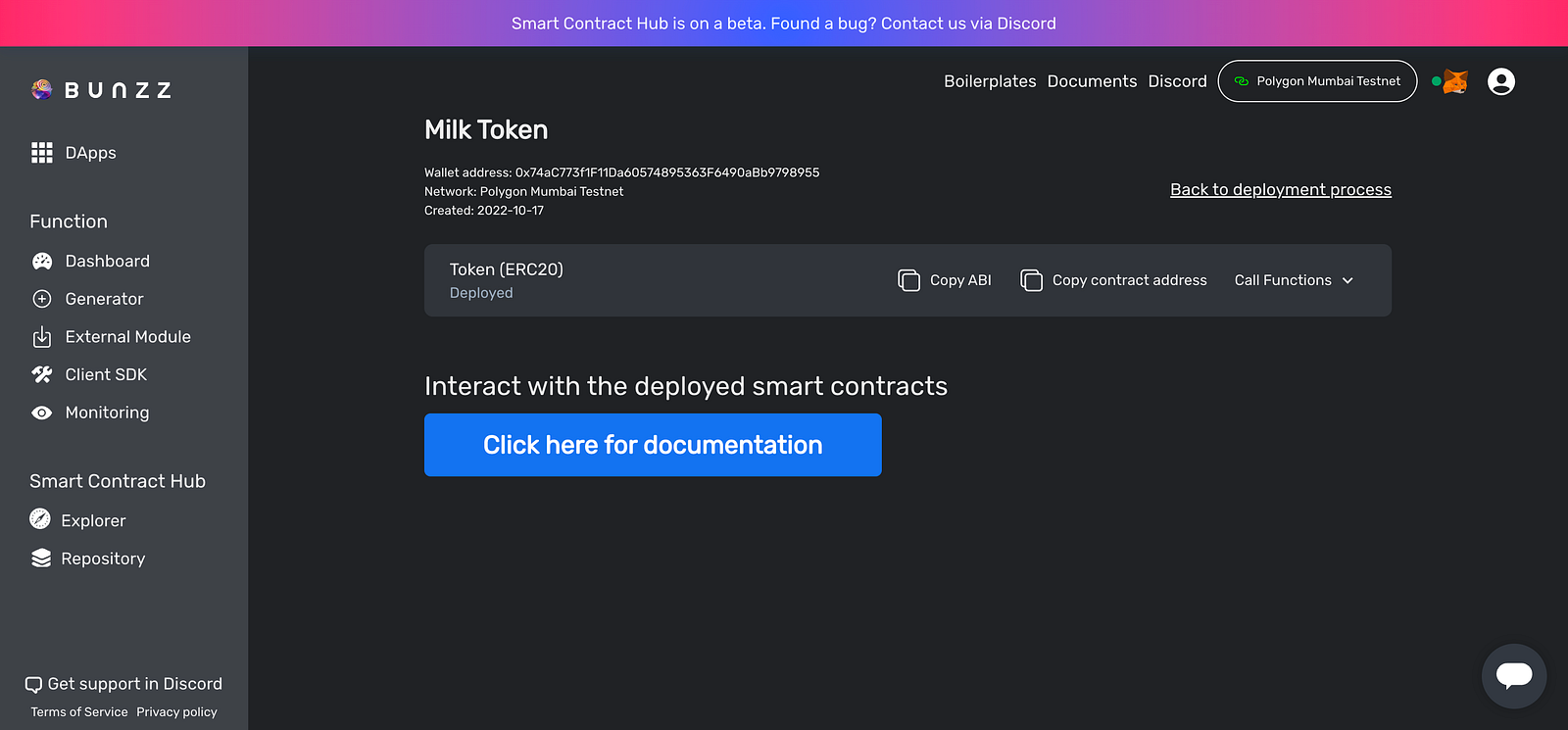
Now, proceed to check Polygon Token Tracker and confirm if the new token is really on the Polygon chain.
- Copy the contract address using the copy icon on the dashboard.
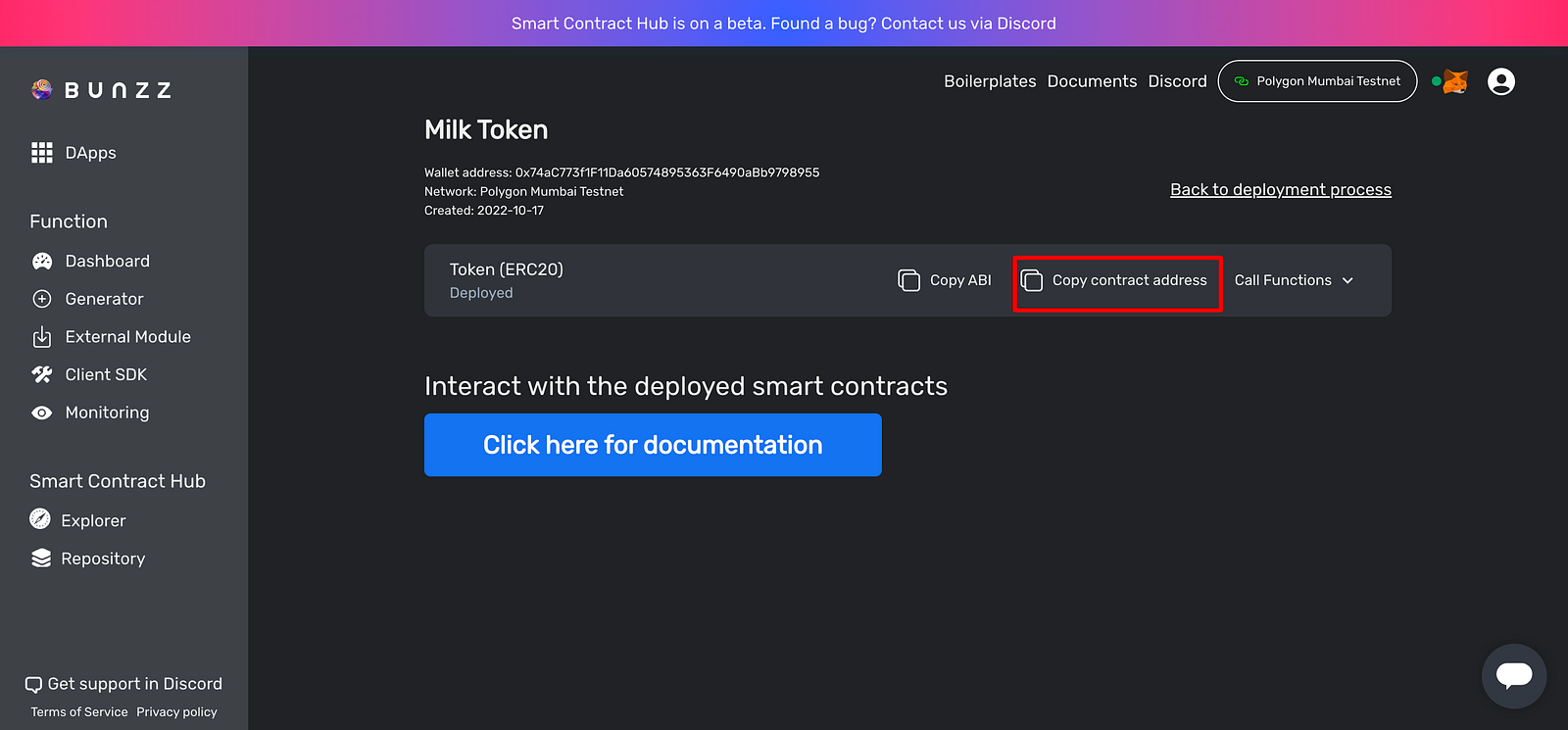
- Paste the contract address on Polygonscan and click on the Find button.
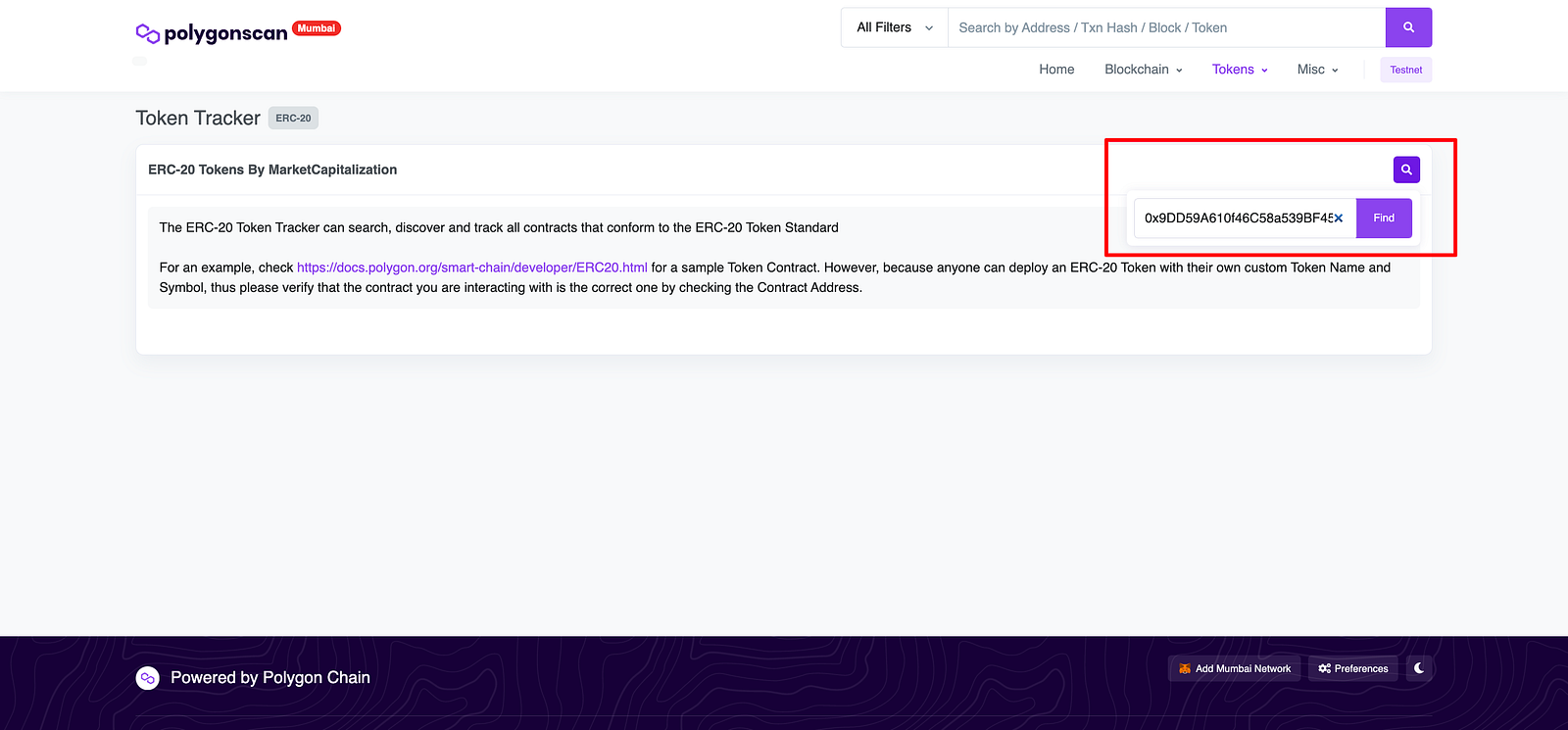
Great!
You can see the token name and symbol we created on the Polygon chain.
Congrats your token has been created!
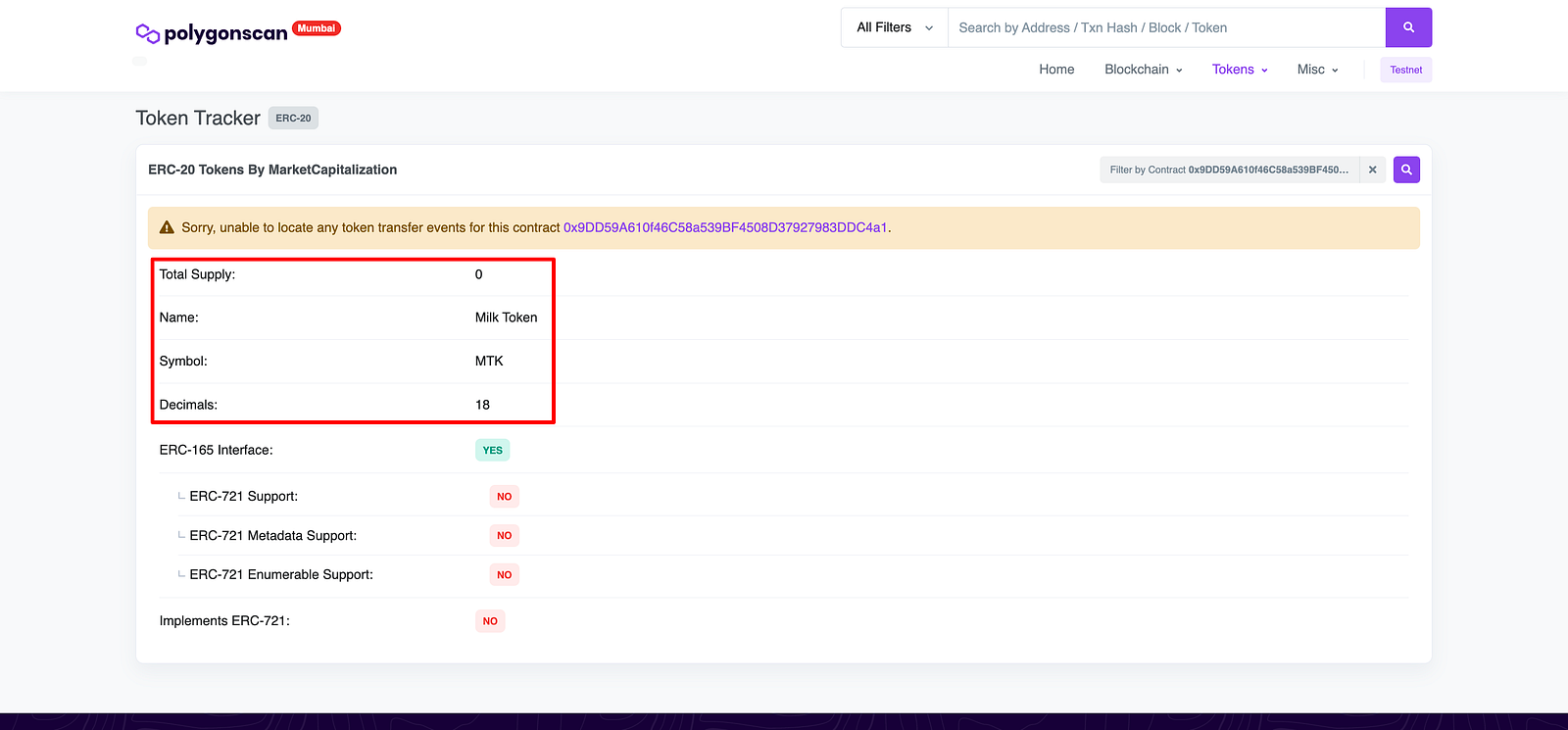
If you look at the image above closely, you’d notice that there’s no supply of Milk Token on the chain. In the next part, we will fix that by minting the Milk Token using ethers.js.
Minting ERC20 Token
To add an initial supply of the new Milk Token, you need to mint it.
What does it mean to mint tokens?
“What is minting? In cryptocurrency, minting is a decentralized method that enables a person to generate a new token without the involvement of a central authority, such as the government or the bank. It can either be a non-fungible token or a crypto coin.” — CoinMarketCap
In this part, you will mint the Milk Token and add supplies of the token into the blockchain for use.
Let’s begin!
Step 1. Create a Frontend Application
To make this guide a little easier, I have provided the React/Ethers.js frontend code for this project. Clone the repo here.
This is what you should see:
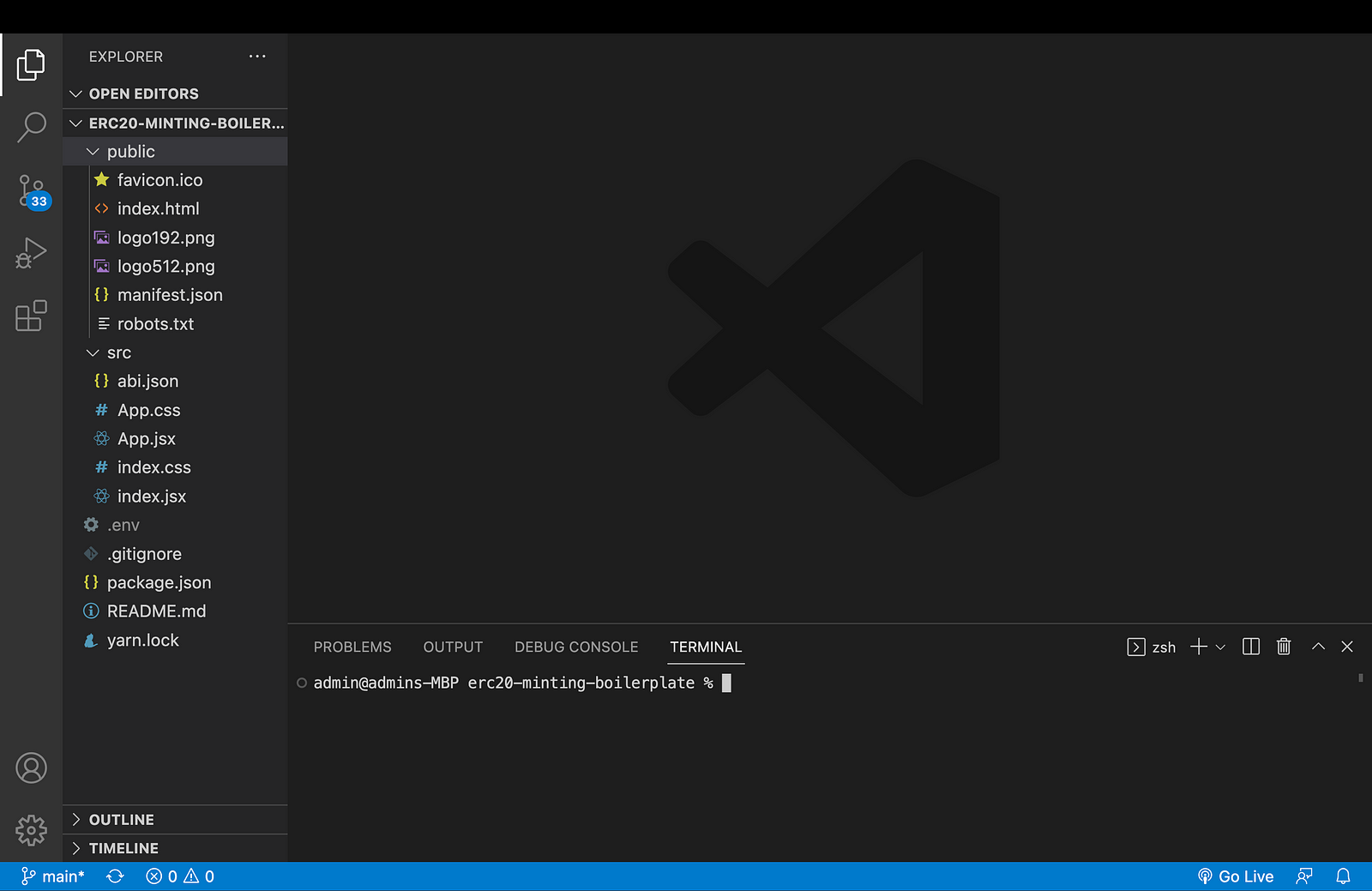
Step 2: Install Dependencies
Next, install all the dependencies using the npm package manager with the command below:
yarn install
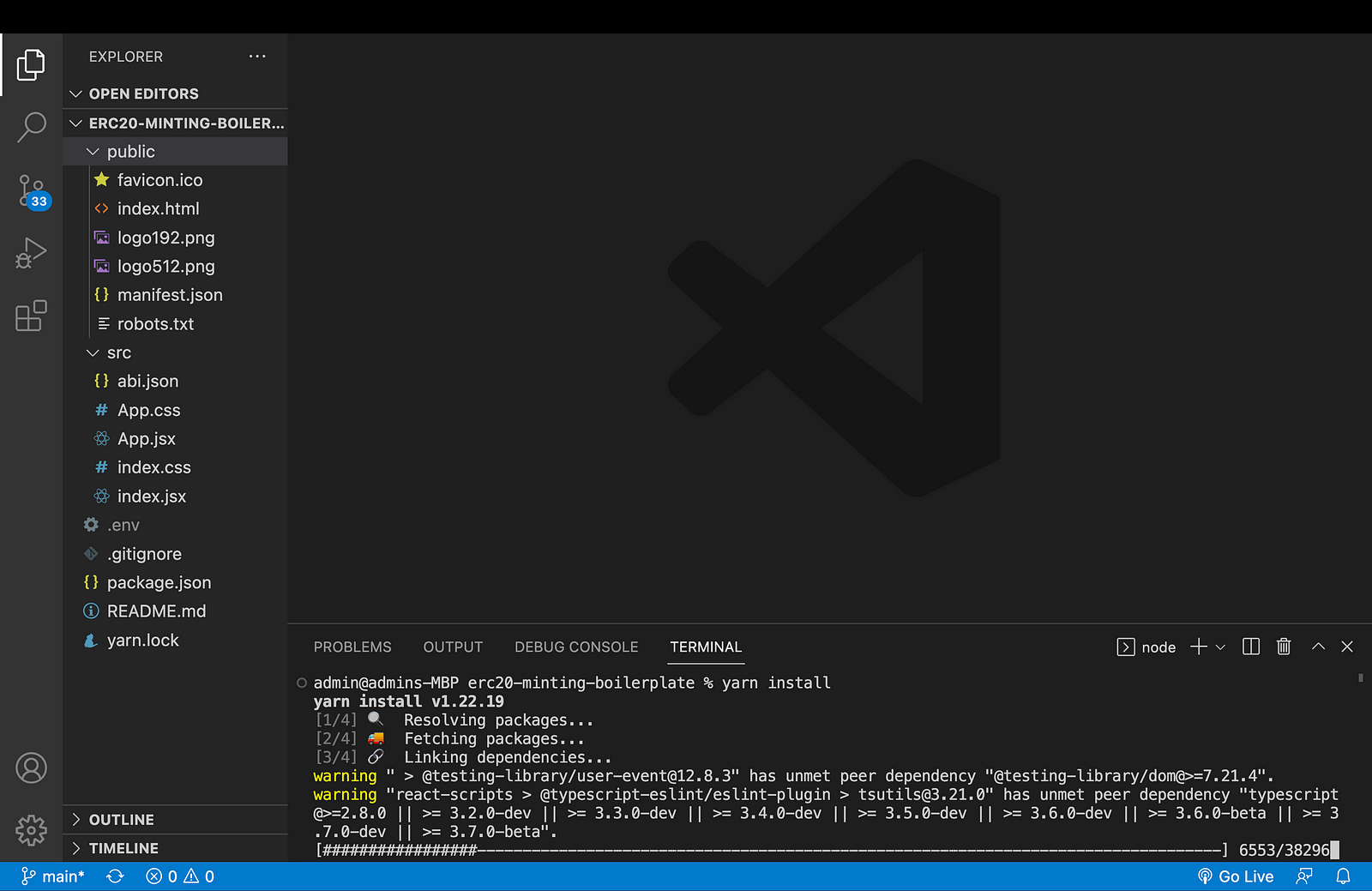
If you do not have yarn already installed on your system, visit this link to install yarn on your system.
Step 3: Copy ABI and Contract Address
Go to your Bunzz dashboard, copy both the ABI and contract address, and save it for later.
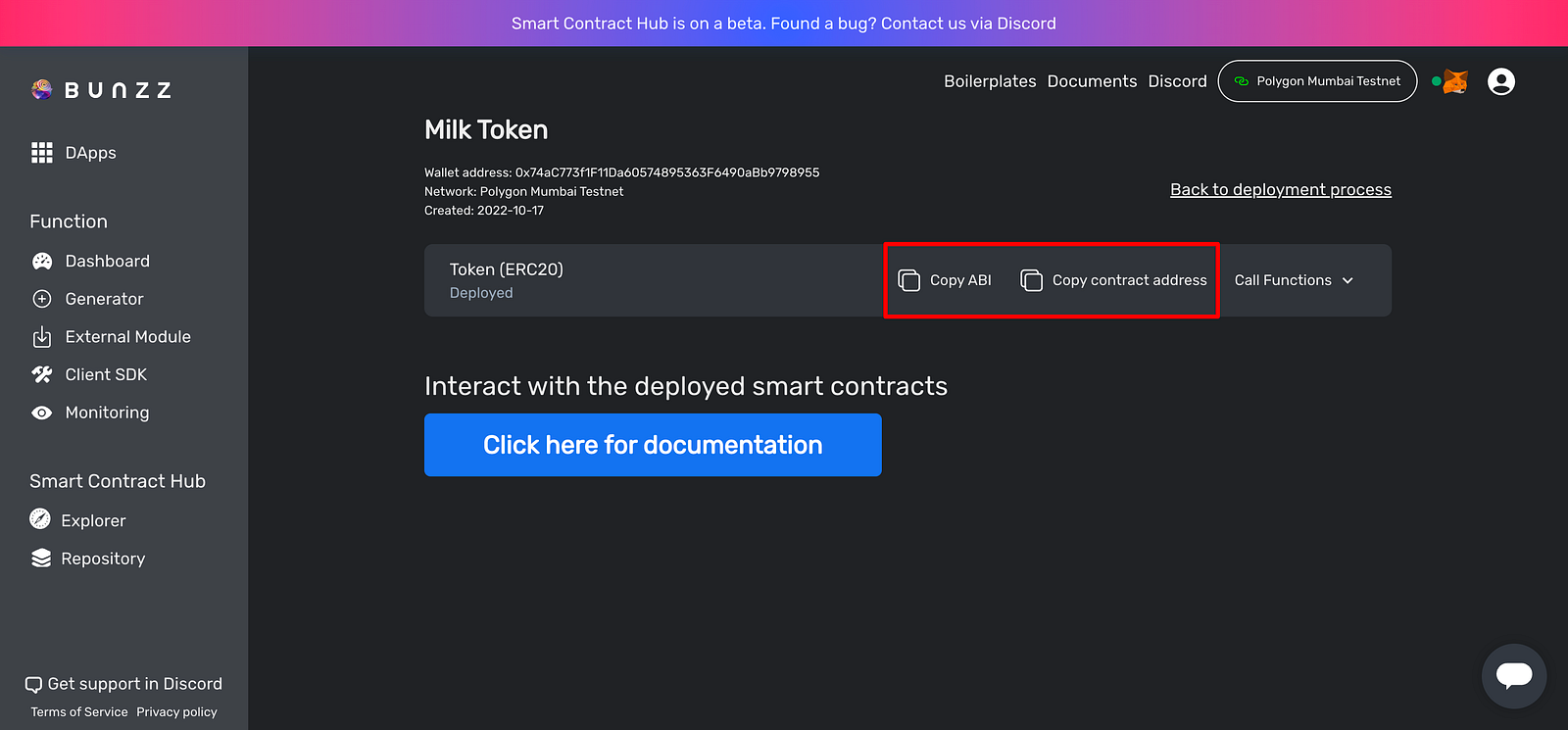
Step 4: Log In to Your MetaMask Account
Since we will need to interact with the Ethereum blockchain, metamask which is a secure wallet will be used. If you are using Chrome or Firefox, Metamask offers an easy-to-use wallet as a Chrome or Firefox extension.
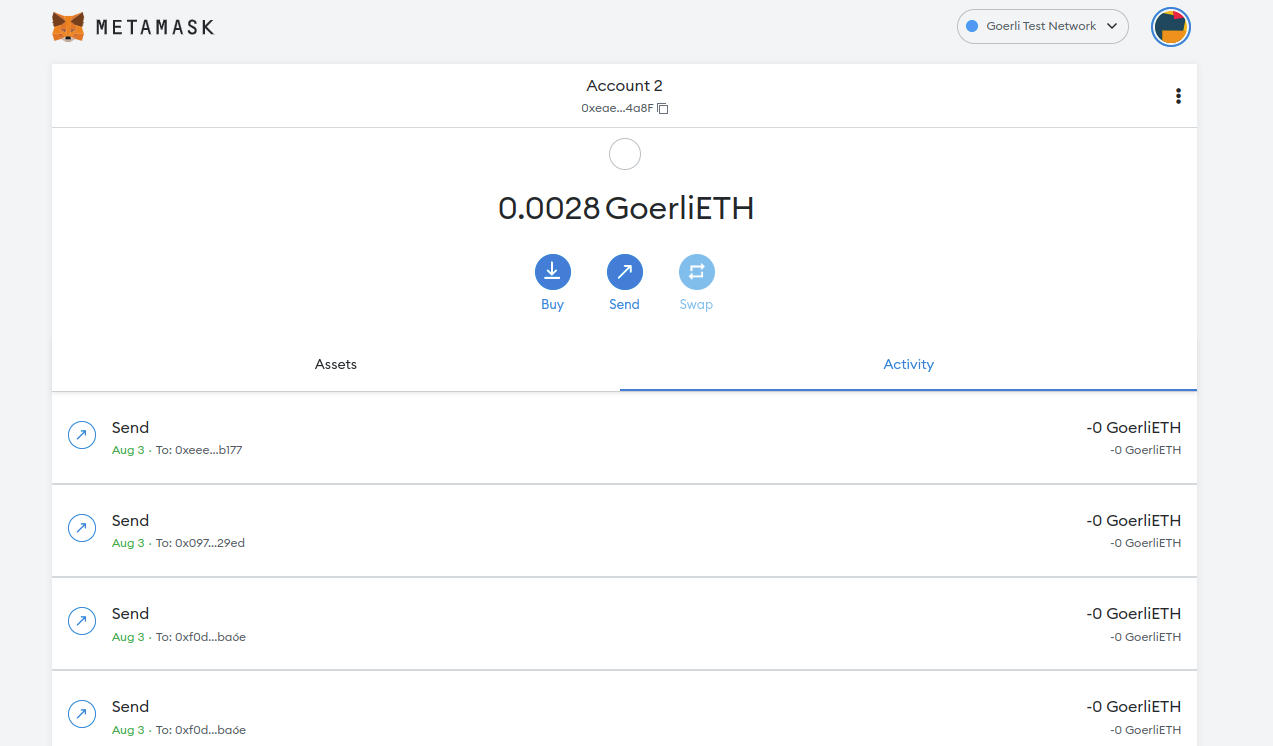
If you do not have MetaMask installed, all you need to do is to download it, install it, and sign up or log in. You can visit this link to download metamask.
Step 5: Start the React App
Start the react server with the command yarn run start on the VS code terminal.
yarn run start
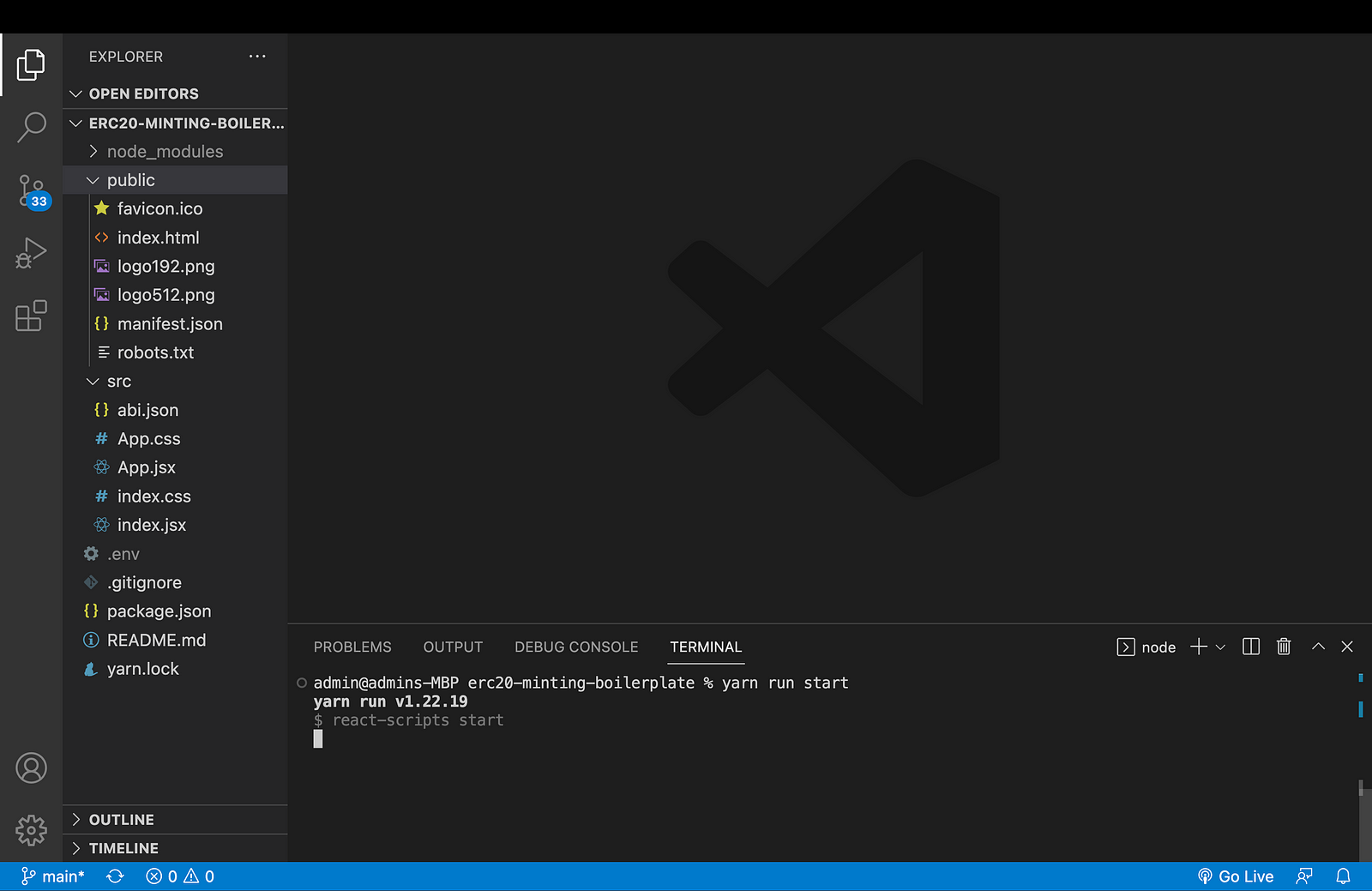
The interface below is what you should see when you start the react server for the first time.
Click the button and connect your metamask wallet to the app.
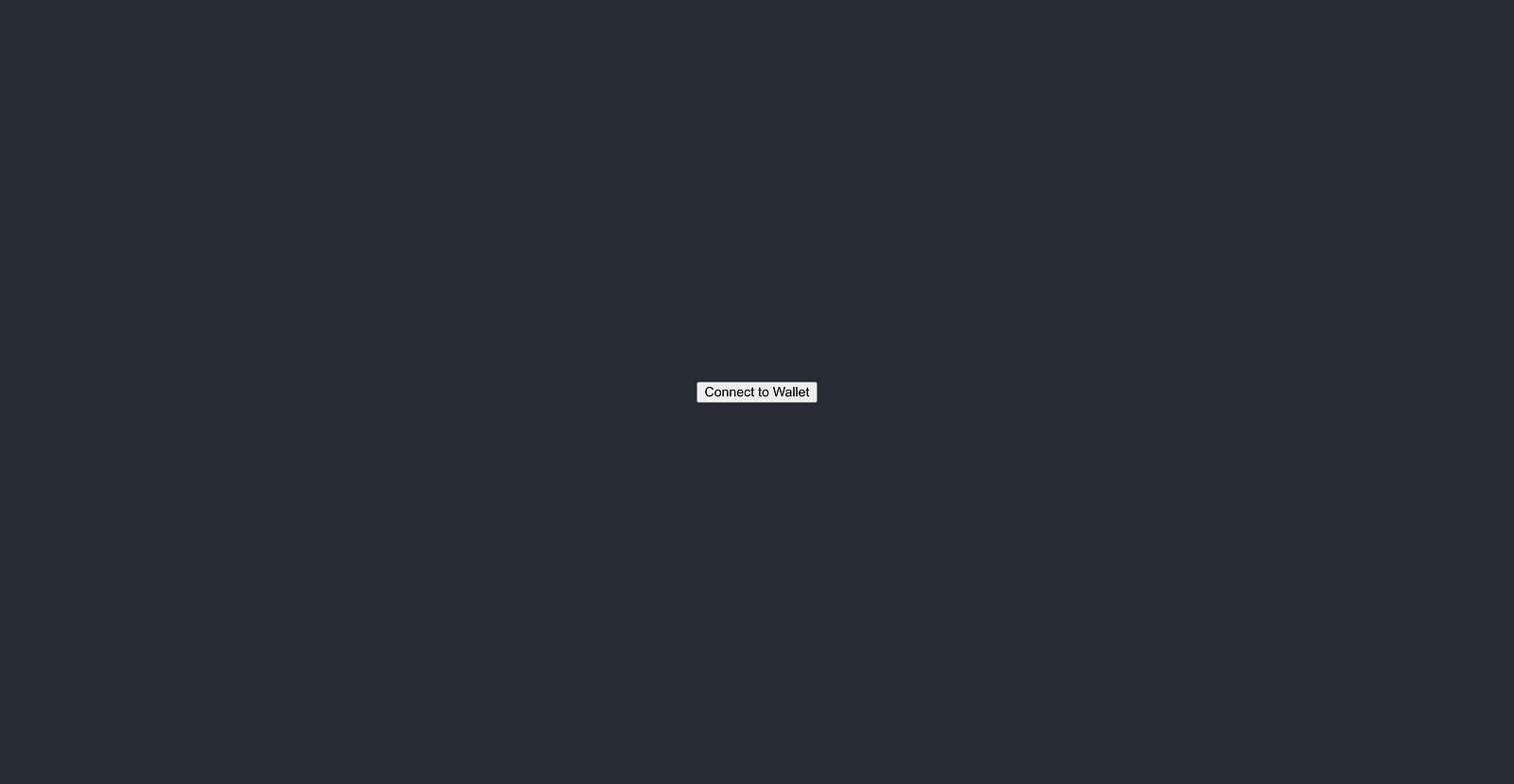
Step 6: Set CHAIN_ID and CONTRACT_ADDRESS
To successfully mint your ERC-20 token using ethers.js, it needs some information about the smart contract you deployed earlier.
Ethers.js will get the information it needs from Bunzz via your YOUR_CHAIN_ID and YOUR_CONTRACT_ADDRESS.
To set the Chain_ID
and CONTRACT_ADDRESS
in the React app project you cloned earlier, create a .env file using this command in the VS code terminal:
touch .env
Replace YOUR_CHAIN_ID and YOUR_CONTRACT_ADDRESS using the values you copied in step 3 and paste them inside the .env file as seen in the image below.
If you don’t know the chain ID, you can search for it on chainlist.org.
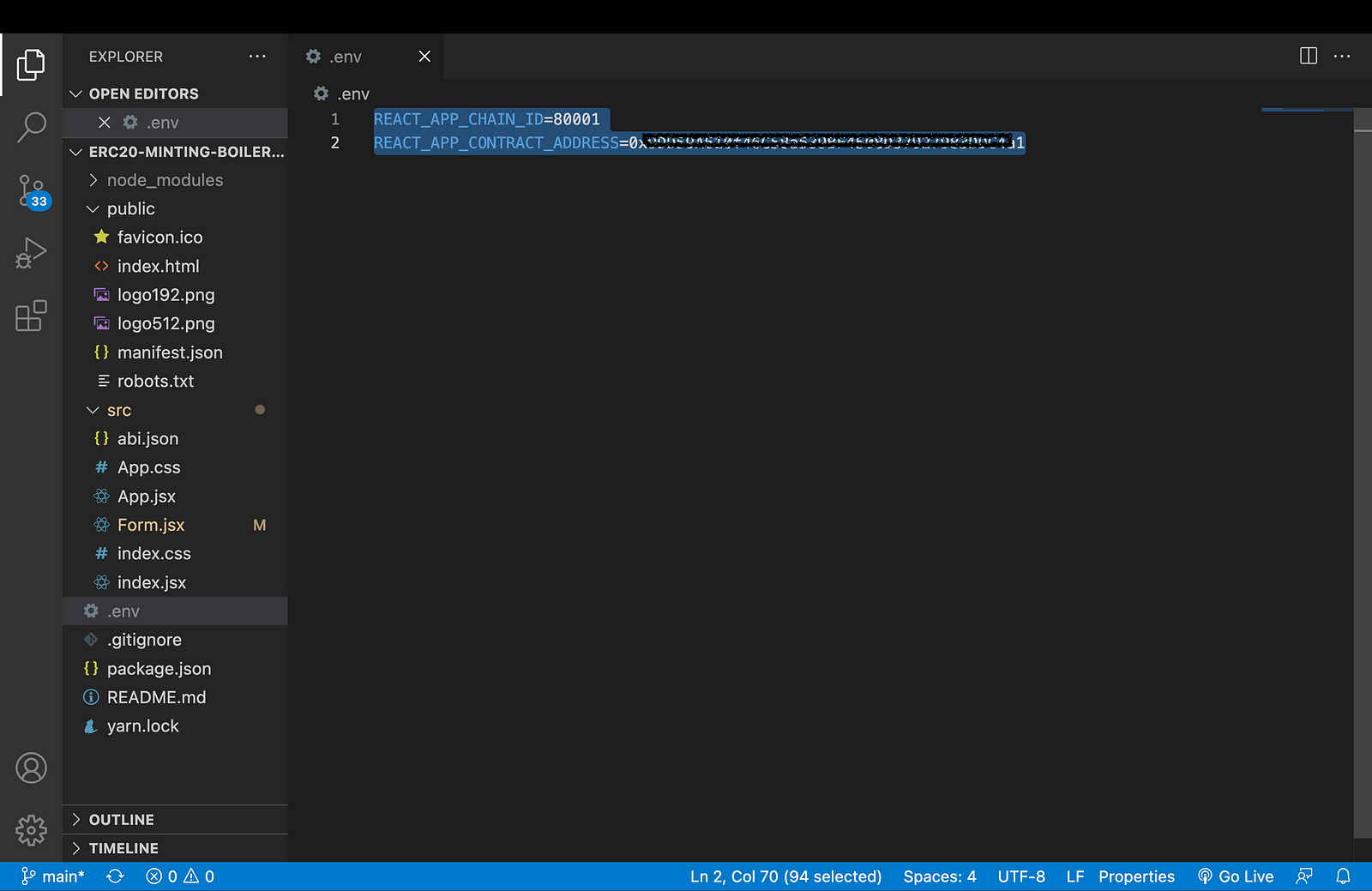
Step 7: Mint Your Token
After you save, start the app again or go to the frontend app if started already and indicate the number of tokens you want to mint, it’s 100 in my case.
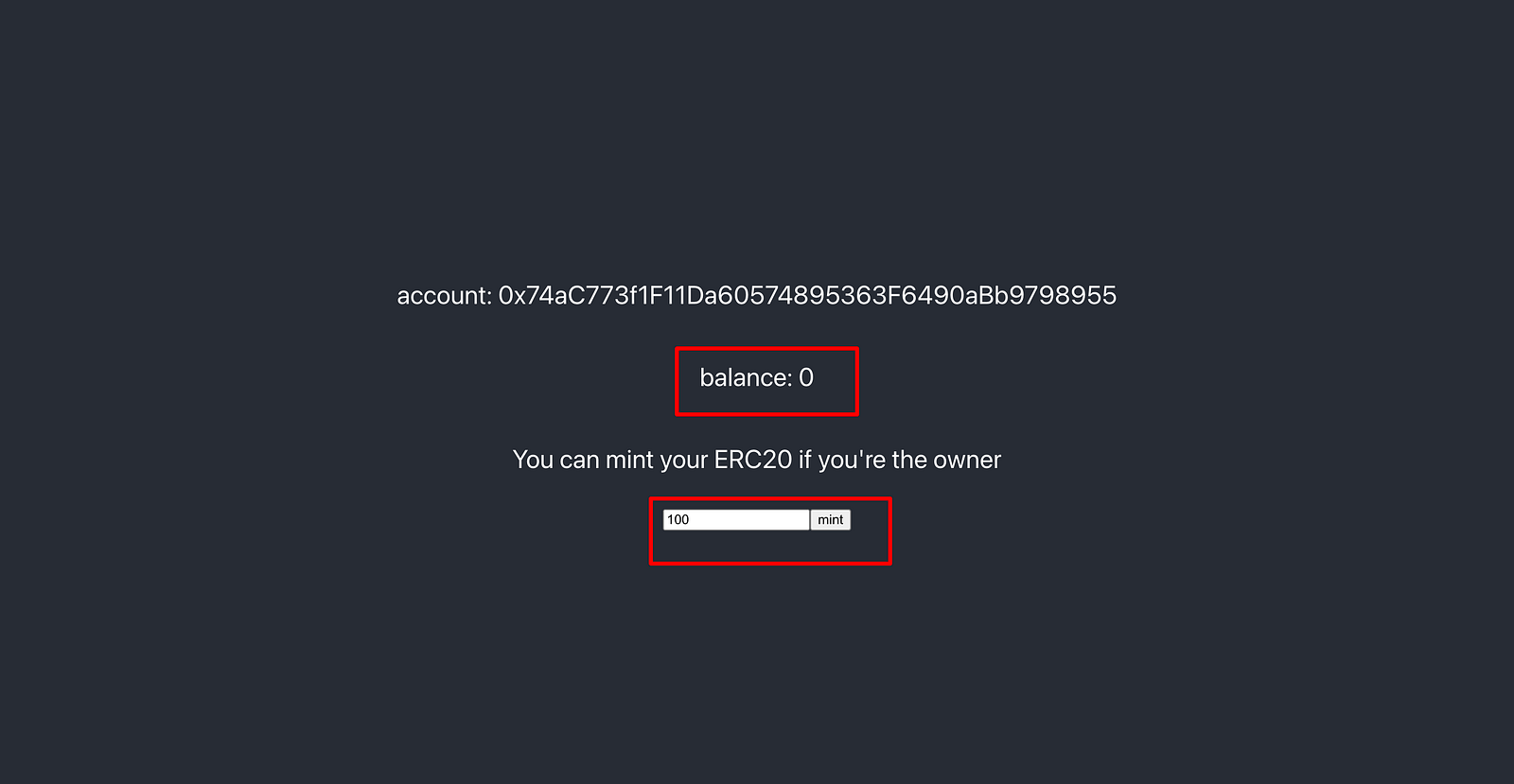
When you click on the mint button, the gas fee required for this transaction then pops up, confirm and that’s all. You have successfully minted your ERC20 token.
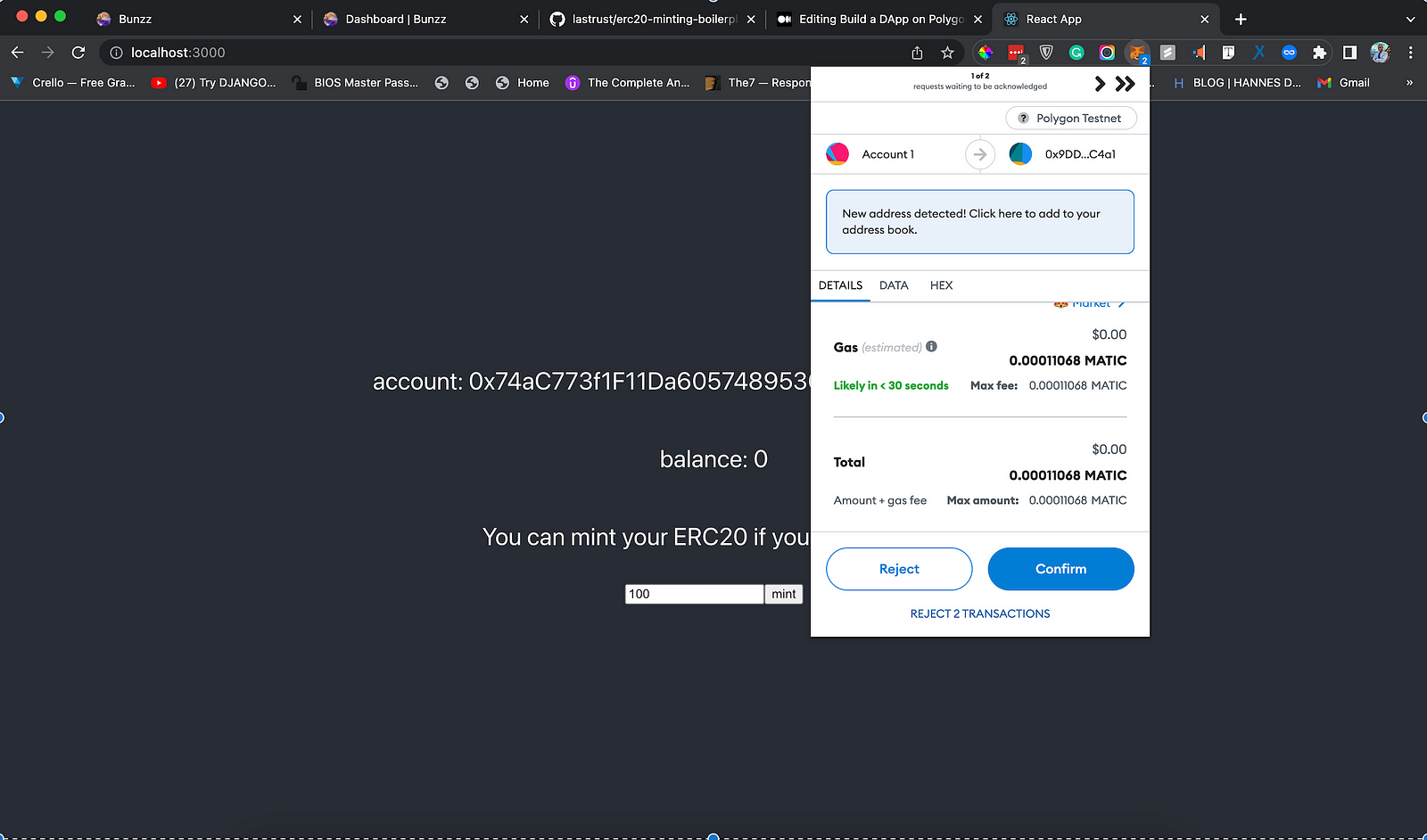
A success message will pop up if minting is done.
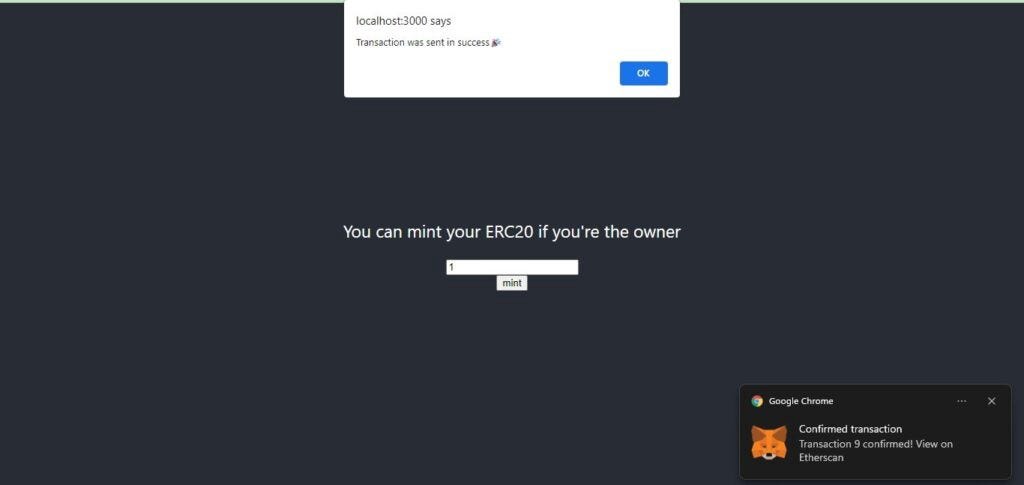
If you refresh the page, you’d observe that the total balance for the token is now 100.
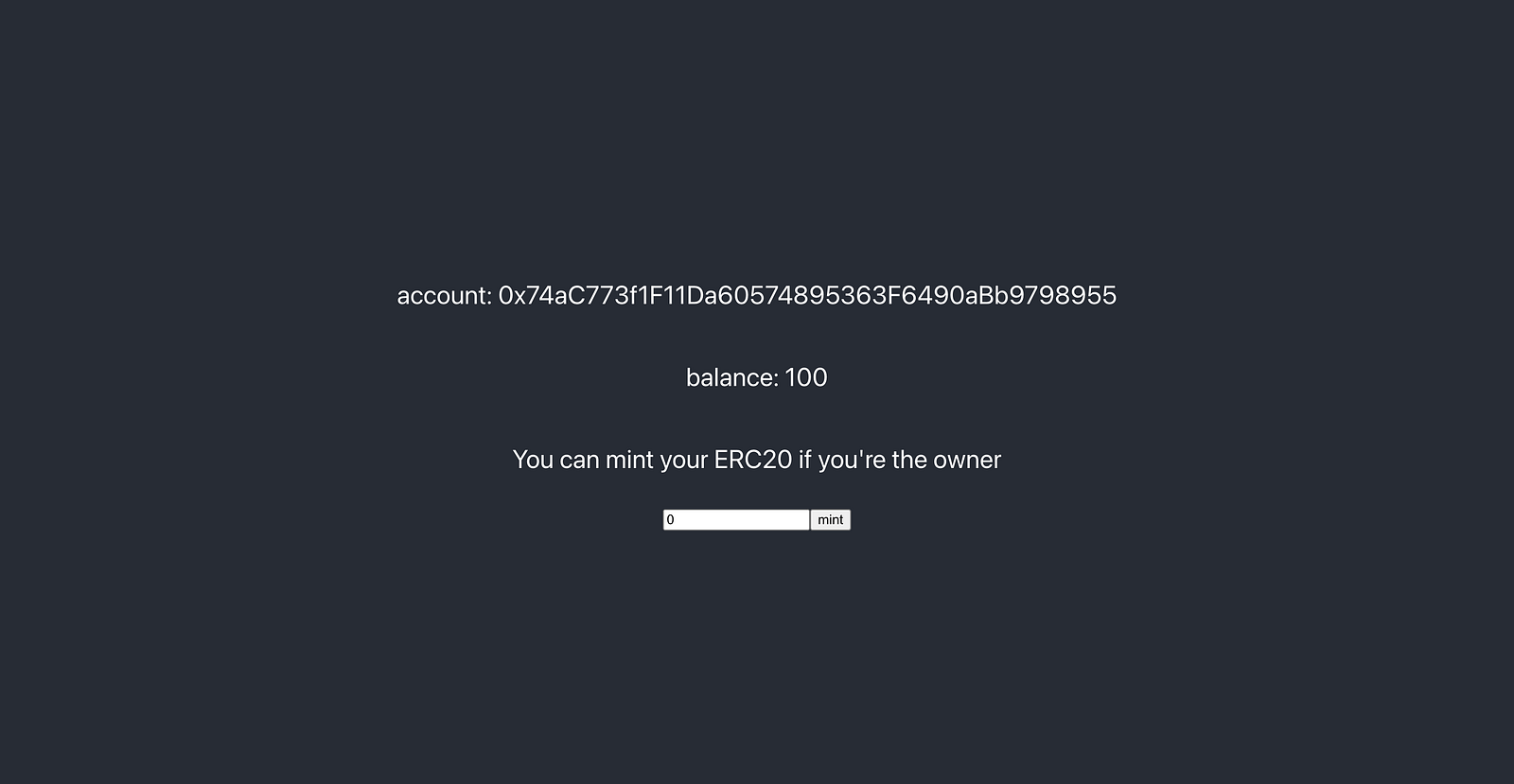
That’s it!
Congratulations! You have now created, deployed, and minted your own ERC-20 token on the polygon network.
To confirm that we have minted the Milk Token, head over to Polygon Token Tracker, paste the token address (as we did towards the end of part 1), and search.
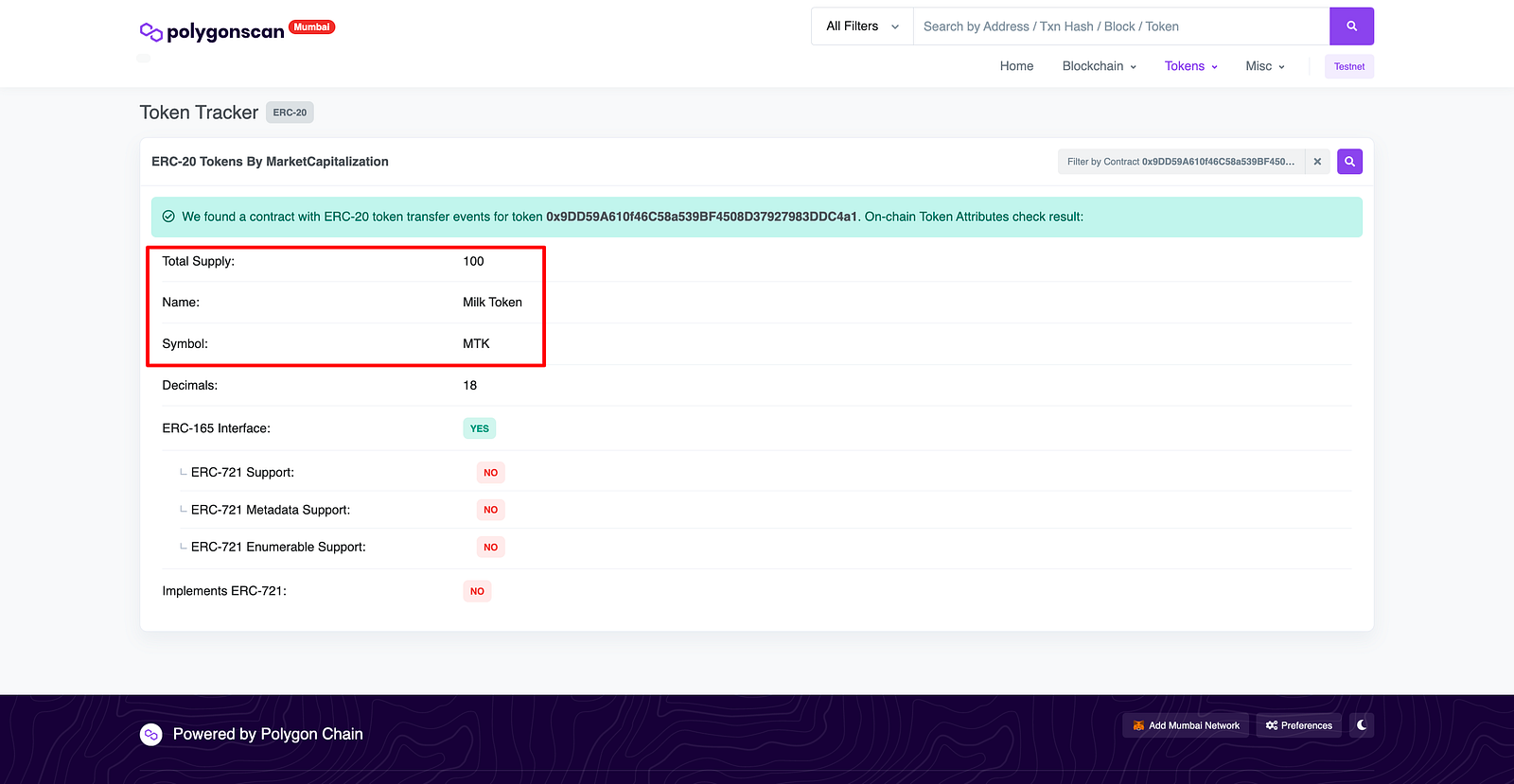
Great!
As you can see in the image above, the token has a total supply of 1 which is what we minted on our React app. You can play around with this and explore how to use this in a real-life scenario.
To know more about the code used to create the smart contract, visit Bunzz documentation and the Bunzz GitHub account.
Conclusion
If you decide to make your own public token, make sure to use this guide only as a starting point. It’s a deep topic that takes a long time to understand fully.
Beyond creating the token, you also need to think about making it a success post-launch and studying other projects and their launches to see what worked well and what didn’t can help with creating your own token.
Want to Connect? If you follow these steps and got stuck, or if you have any questions, feel free to reach out on Discord or send a DM on Twitter.